Publishing Content
Content can be published automatically through the Cortex Click GitHub app or by using the SDK/API to write custom publication scripts.
Publishing to GitHub
The Cortex Click GitHub app enables one-click publishing from within the Cortex Click app to publish any piece of generated content in a pull request.
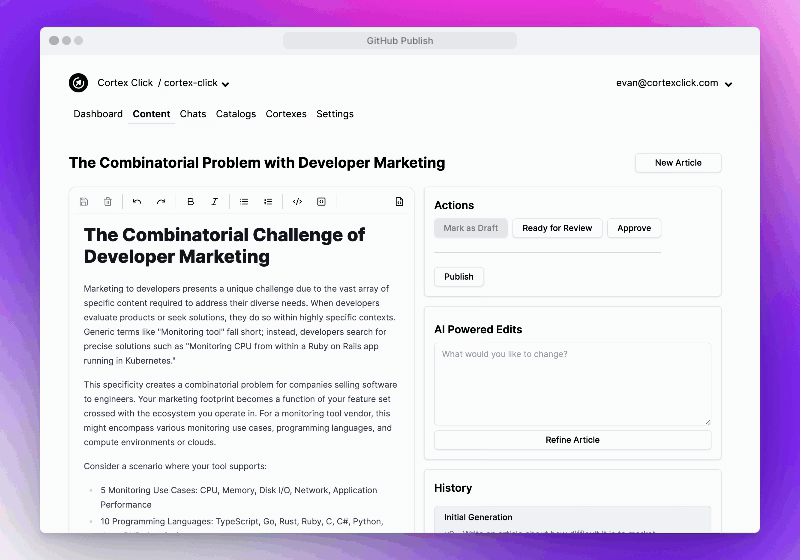
Publishing via the SDK
The SDK can be used to automate publishing.
It is recommended that you take advantage of the content status
field to mark production-ready content as PUBLISHED
.
From there a simple script can be run as a build step within your website:
- Use
client.listContent
to iterate through all pages of content withstatus: "PUBLISHED"
- Retrieve each piece of content
- Write the
content
field to a file in the appropriate location
const client = new CortexClient({
org: "your-org-name",
accessToken: process.env.CORTEX_ACCESS_TOKEN,
});
// update destinations to match local desired directory structure
const publicationConfig = [
{ cortexName: "blog-writer", destination: ["content", "blog"] },
{
cortexName: "tutorial-writer",
destination: ["content", "learn", "tutorials"],
},
];
// utility to create file names from titles
const slugify = (title: string) => {
return title
.toLowerCase() // Convert to lowercase
.replace(/[^\w\s-]/g, "") // Remove special characters except hyphens and whitespace
.replace(/\s+/g, "-") // Replace spaces with hyphens
.replace(/-+/g, "-"); // Replace multiple hyphens with a single hyphen
};
// utility to page through all published content from a cortex and write it to local files
const renderContent = async (cortexName: string, destination: string[]) => {
let page = client.listContent({
cortexname,
status: ContentStatus.Published,
});
// continue paging until we get an empty result
while (page.content.length) {
// iterate through each list result item and retrieve the full content
for (const listResult of page.content) {
const content = await listResult.Content();
const slug = slugify(content.title);
const fpath = path.join(...destination, `${slug}.md`);
// write content to local file
fs.writeFileSync(fpath, content.content);
}
page = await page.nextPage();
}
};
for (const config of publicationConfig) {
const { cortexName, destination } = config;
await renderContent(cortexName, destination);
}